No Plugins Required
Adding JavaScript to WordPress can seem like a headache, but it doesn’t need to be. Unlike what some articles lead you to believe, I found that it can even be done without any plugins.
Sample Embed Example:
Step by Step
Step 1: Add a Custom HTML
block.
From the editing dashboard for your page, add a new block.
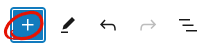
Choose the Custom HTML
block from the menu.
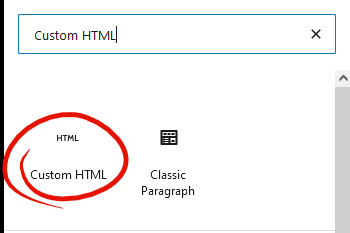
Step 2: Add code to the Custom HTML
block.
In your new HTML block, paste your code in the following order:
- CSS: wrapped in
<style> </style>
tags. - HTML: plain, not wrapped in anything.
- Javascript Script: wrapped in
<script> </script>
tags.
Step 2.5: Short Example
Paste all the code into a single Custom HTML
block for it to render in your page.
Sample typing effect.
CSS
<style>
body, html {
margin: 0;
height: 100%;
text-align: center;
font-family: 'Oxygen Mono', monospace;
color: #999;
}
h1 {
text-transform: uppercase;
letter-spacing: 1pt;
font-size: 30pt;
margin-bottom: 15px;
}
p {
text-align: left;
margin: 0;
text-transform: lowercase;
font-size: 10pt;
font-weight: 900;
width: 50%;
display: none;
}
#table {
display: table;
width: 100%;
height: 100%;
background-color: #e5e5e5;
}
#centeralign {
display: table-cell;
vertical-align: middle;
}
</style><style>
body, html {
margin: 0;
height: 100%;
text-align: center;
font-family: 'Oxygen Mono', monospace;
color: #999;
}
h1 {
text-transform: uppercase;
letter-spacing: 1pt;
font-size: 30pt;
margin-bottom: 15px;
}
p {
text-align: left;
margin: 0;
text-transform: lowercase;
font-size: 10pt;
font-weight: 900;
width: 50%;
display: none;
}
#table {
display: table;
width: 100%;
height: 100%;
background-color: #e5e5e5;
}
#centeralign {
display: table-cell;
vertical-align: middle;
}
</style>
HTML
<div id="table">
<div id="centeralign">
<h1>Sample typing effect.</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec ante arcu, dignissim non risus id, posuere efficitur felis. Vestibulum arcu diam, semper non ipsum quis, dictum ultricies diam. Suspendisse vel luctus sapien. Mauris tristique condimentum velit tincidunt pharetra. Curabitur ut lectus eleifend, malesuada lorem eget, consectetur augue. Nunc scelerisque nisi in lacus eleifend eleifend. Praesent blandit ex at nunc maximus, ut sodales ante auctor. Nunc elementum eros sit amet malesuada facilisis. Morbi eget elit consequat, sodales urna in, lobortis nisi. Morbi dapibus velit eu mattis bibendum. Nulla et nisi eget turpis vulputate suscipit eu nec nunc. Pellentesque ut pulvinar quam.</p>
</div>
</div>
Javascript
<script>
function typeEffect(element, speed) {
var text = element.innerHTML;
element.innerHTML = "";
var i = 0;
var timer = setInterval(function() {
if (i < text.length) {
element.append(text.charAt(i));
i++;
} else {
clearInterval(timer);
}
}, speed);
}
// application
var speed = 75;
var h1 = document.querySelector('h1');
var p = document.querySelector('p');
var delay = h1.innerHTML.length * speed + speed;
// type affect to header
typeEffect(h1, speed);
// type affect to body
setTimeout(function(){
p.style.display = "inline-block";
typeEffect(p, speed);
}, delay);
</script>
I hope my short post helped you out. If any questions come up, feel free to leave a comment!